This is a quick walkthrough of getting your first web service up and running whilst having a look at the how some of the different components work.
Step 1: Install the x dotnet tool​
First we want to install the x dotnet tool:
dotnet tool install --global x
The dotnet tools are ServiceStack's versatile companion giving you quick access to a lot of its high-level features including generating mobile, web & desktop DTOs with Add ServiceStack Reference generating gRPC Clients and proto messages, quickly apply gists to your project enabled by ServiceStack's effortless no-touch Modular features, command-line API access, it even includes a lisp REPL should you need to explore your remote .NET Apps in real-time.
Step 2: Selecting a template​
Importantly, the dotnet tools lets you create .NET 6, .NET Framework and ASP.NET Core on .NET Framework projects. Unless you're restricted to working with .NET Framework you'll want to start with a .NET 6 project template, for this example we'll start with the Empty web template which implicitly uses the folder name for the Project Name:
x new web WebApp
Step 3: Run your project​
Press Ctrl+F5
to run your project!
You should see an already working API integration using @servicestack/client library to call your App's JavaScript DTOs and links to calling your API from API Explorer:
Watched builds​
A recommended alternative to running your project from your IDE is to run a watched build using dotnet watch
from a terminal:
dotnet watch
Where it will automatically rebuild & restart your App when it detects any changes to your App's source files.
How does it work?​
Now that your new project is running, let's have a look at what we have. The template comes with a single web service route which comes from the Request DTO (Data Transfer Object) which is located in the Hello.cs file:
[Route("/hello/{Name}")]
public class Hello : IReturn<HelloResponse>
{
public string Name { get; set; }
}
public class HelloResponse
{
public string Result { get; set; }
}
The Route
attribute is specifying what path /hello/{Name}
where {Name}
binds its value to the public string property of Name.
Let's access the route to see what comes back. Go to the following URL in your address bar:
/hello/world
You will see a snapshot of the Result in a HTML response format. To change the return format to Json, simply add ?format=json
to the end of the URL. You'll learn more about formats, endpoints (URLs, etc) when you continue reading the documentation.
If we go back to the solution and find the WebApplication1.ServiceInterface and open the MyServices.cs file, we can have a look at the code that is responding to the browser, giving us the Result back.
public class MyServices : Service
{
public object Any(Hello request)
{
return new HelloResponse { Result = $"Hello, {request.Name}!" };
}
}
If we look at the code above, there are a few things to note. The name of the method Any
means the server will run this method for any of the valid HTTP Verbs. Service methods are where you control what returns from your service.
Step 4: Exploring the ServiceStack Solution​
The Recommended structure below is built into all ServiceStackVS VS.NET Templates where creating any new ServiceStack project will create a solution with a minimum of 4 projects below ensuring ServiceStack solutions starts off from an optimal logical project layout, laying the foundation for growing into a more maintainable, cohesive and reusable code-base:
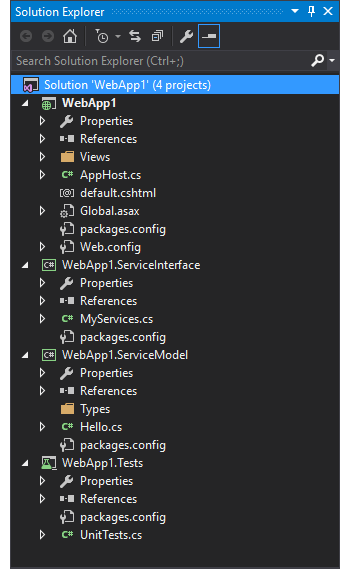
Host Project​
The Host project contains your AppHost which references and registers all your App's concrete dependencies in its IOC and is the central location where all App configuration and global behavior is maintained. It also references all Web Assets like Razor Views, JS, CSS, Images, Fonts, etc. that's needed to be deployed with the App. The AppHost is the top-level project which references all dependencies used by your App whose role is akin to an orchestrator and conduit where it decides what functionality is made available and which concrete implementations are used. By design it references all other (non-test) projects whilst nothing references it and as a goal should be kept free of any App or Business logic.
ServiceInterface Project​
The ServiceInterface project is the implementation project where all Business Logic and Services live which typically references every other project except the Host projects. Small and Medium projects can maintain all their implementation here where logic can be grouped under feature folders. Large solutions can split this project into more manageable cohesive and modular projects which we also recommend encapsulates any dependencies they might use.
ServiceModel Project​
The ServiceModel Project contains all your Application's DTOs which is what defines your Services contract, keeping them isolated from any Server implementation is how your Service is able to encapsulate its capabilities and make them available behind a remote facade. There should be only one ServiceModel project per solution which contains all your DTOs and should be implementation, dependency and logic-free which should only reference the impl/dep-free ServiceStack.Interfaces.dll contract assembly to ensure Service contracts are decoupled from its implementation, enforces interoperability ensuring that your Services don't mandate specific client implementations and will ensure this is the only project clients need to be able to call any of your Services by either referencing the ServiceModel.dll directly or downloading the DTOs from a remote ServiceStack instance using Add ServiceStack Reference:
Test Project​
The Unit Test project contains all your Unit and Integration tests. It's also a Host project that typically references all other non-Host projects in the solution and contains a combination of concrete and mock dependencies depending on what's being tested. See the Testing Docs for more information on testing ServiceStack projects.
Learn ServiceStack Guide​
If you're new to ServiceStack we recommend stepping through ServiceStack's Getting Started Guide to get familiar with the basics.
API Client Examples​
jQuery Ajax​
ServiceStack's clean Web Services makes it simple and intuitive to be able to call ServiceStack Services from any ajax client, e.g. from a traditional Bootstrap Website using jQuery:
<input class="form-control" id="Name" type="text" placeholder="Type your name">
<p id="result"></p>
<script>
$('#Name').keyup(function () {
let name = $(this).val()
$.getJSON('/hello/' + name)
.success(function (response) {
$('#result').html(response.Result)
})
})
</script>
Rich JsonApiClient & Typed DTOs​
The modern recommended alternative to jQuery that works in all modern browsers is using your APIs built-in JavaScript typed DTOs with the @servicestack/client library from a JavaScript Module.
We recommend using an importmap to specify where @servicestack/client should be loaded from, e.g:
<script async src="https://ga.jspm.io/npm:es-module-shims@1.6.3/dist/es-module-shims.js"></script><!--safari-->
<script type="importmap">
{
"imports": {
"@servicestack/client":"https://unpkg.com/@servicestack/client@2/dist/servicestack-client.mjs"
}
}
</script>
This lets us reference the @servicestack/client package name in our source code instead of its physical location:
<input type="text" id="txtName">
<div id="result"></div>
<script type="module">
import { JsonServiceClient, $1, on } from '@servicestack/client'
import { Hello } from '/types/mjs'
const client = new JsonServiceClient()
on('#txtName', {
async keyup(el) {
const api = await client.api(new Hello({ name:el.target.value }))
$1('#result').innerHTML = api.response.result
}
})
</script>
Enable static analysis and intelli-sense​
For better IDE intelli-sense during development, save the annotated Typed DTOs to disk with the x dotnet tool:
x mjs
Then reference it instead to enable IDE static analysis when calling Typed APIs from JavaScript:
import { Hello } from '/js/dtos.mjs'
client.api(new Hello({ name }))
To also enable static analysis for @servicestack/client, install the dependency-free library as a dev dependency:
npm install -D @servicestack/client
Where only its TypeScript definitions are used by the IDE during development to enable its type-checking and intelli-sense.
Rich intelli-sense support​
Where you'll be able to benefit from rich intelli-sense support in smart IDEs like Rider for both the client library:
As well as your App's server generated DTOs:
So even simple Apps without complex bundling solutions or external dependencies can still benefit from a rich typed authoring experience without any additional build time or tooling complexity.
Create Empty ServiceStack Apps​
If you don't have the x dotnet tool installed, the quickest way to create a ServiceStack App is to download your preferred template from:
Alternatively if you're creating multiple projects we recommend installing the x dotnet tool which can create every project template:
dotnet tool install --global x
Empty Projects​
There are a few different ways you can create empty ServiceStack projects ordered by their level of emptiness.
To write a minimal .NET 6 Web App to your current directory, mix in the init gist files to your current directory:
x mix init
To create an empty Single Project Template solution use the empty template:
x new empty ProjectName
To create an empty 4 Project solution that adopts ServiceStack's recommended project structure, use the web template:
x new web ProjectName
INFO
You can omit the ProjectName in all above examples to use the Directory Name as the Project Name
Empty F# Template​
Like C# init there's also init-fsharp gist to create an empty F# Web App:
x mix init-fsharp
Empty VB .NET Template​
And init-vb to create an empty VB .NET Web App:
x mix init-vb
Any TypeScript or JavaScript Web, Node.js or React Native App​
The same TypeScript JsonServiceClient can also be used in more sophisticated JavaScript Apps like React Native to Node.js Server Apps such as this example using TypeScript & Vue Single-File Components:
<template>
<div v-if="api.error" class="ml-2 text-red-500">{{ error.message }}</div>
<div v-else class="ml-3 mt-2 text-2xl">{{ api.loading ? 'Loading...' : api.response.result }}</div>
</template>
<script setup lang="ts">
import { JsonServiceClient } from "@servicestack/client"
import { Hello } from "@/dtos"
const props = defineProps<{ name: string }>()
const client = new JsonServiceClient()
const api = client.api(new Hello({ name: props.name }))
</script>
Compare and contrast with other major SPA JavaScript Frameworks:
- Vue 3 HelloApi.mjs
- Vue SSG using swrClient
- Next.js with swrClient
- React HelloApi.tsx
- Angular HelloApi.ts
- Svelte Home.svelte
Web, Mobile and Desktop Apps​
Use Add ServiceStack Reference to enable typed integrations for the most popular languages to develop Web, Mobile & Desktop Apps.
Full .NET Project Templates​
The above init
projects allow you to create a minimal web app, to create a more complete ServiceStack App with the recommended project structure, start with one of our C# project templates instead:
C# Project Templates Overview​
Simple, Modern Razor Pages & MVC Vue 3 Tailwind Templates​
The new Tailwind Razor Pages & MVC Templates enable rapid development of Modern Tailwind Apps without the pitfalls plaguing SPAs:
All Vue Tailwind templates are pre-configured with our rich Vue 3 Tailwind Components library for maximum productivity:
Advanced JAMStack Templates​
For more sophisticated Apps that need the best web tooling that npm can offer checkout our JAMStack Vite Vue & SSG templates:
Or if you prefer Modern React Apps checkout the Next.js template:
For Blazor WASM and Server checkout our comprehensive Blazor projects & Tailwind components.
Integrated in Major IDEs and popular Mobile & Desktop platforms​
ServiceStack Services are also easily consumable from all major Mobile and Desktop platforms including native iPhone and iPad Apps on iOS with Swift, Mobile and Tablet Apps on Android with Java or Kotlin, OSX Desktop Applications as well as targeting the most popular .NET Mobile and Desktop platforms including Xamarin.iOS, Xamarin.Android, Windows Store, WPF and WinForms.
Instant Client Apps​
Generate working native client apps for your live ServiceStack services, in a variety of languages, instantly with our free managed service.
This tool enables your developers, and even your customers, to open a working example native application straight from the web to their favorite IDE.
Fundamentals - AppHost and Configuration​
Walk through configuring your ServiceStack Application's AppHost
:
Community Resources​
- Creating A Simple Service Using ServiceStack by Shashi Jeevan
- Introducing ServiceStack by @dotnetcurry
- Create web services in .NET in a snap with ServiceStack by @techrepublic
- How to build web services in MS.Net using ServiceStack by @kishoreborra
- Getting started with ServiceStack – Creating a service
- ServiceStack Quick Start by @aarondandy
- Getting Started with ASP.NET MVC, ServiceStack and Bootstrap by @pluralsight
- Building Web Applications with Open-Source Software on Windows by @pluralsight
- ServiceStack the way I like it by @tonydenyer
- Generating a RESTful Api and UI from a database with LLBLGen by @mattjcowan
- ServiceStack: Reusing DTOs by @korneliuk
- ServiceStack, Rest Service and EasyHttp by @chrissie1
- Building a Web API in SharePoint 2010 with ServiceStack
- REST Raiding. ServiceStack by Daniel Gonzalez
- JQueryMobile and ServiceStack: EventsManager tutorial / Part 3 by Kyle Hodgson
- Like WCF: Only cleaner! by Kyle Hodgson
- ServiceStack I heart you. My conversion from WCF to SS by @philliphaydon
- ServiceStack vs WCF Data Services
- Buildiing a Tridion WebService with jQuery and ServiceStack by @robrtc
- Anonymous type + Dynamic + ServiceStack == Consuming cloud has never been easier by @ienablemuch
- Handful of examples of using ServiceStack based on the ServiceStack.Hello Tutorial by @82unpluggd